Check If An Object Has An Attribute In Python - Quick Guide
In Python, the `hasattr()` function is used to check if an object has a specific attribute. It takes two arguments: the object to be checked and the attribute name to be checked for. The function returns `True` if the object has the specified attribute, and `False` otherwise.
The `hasattr()` function can be used to check for both instance attributes and class attributes. Instance attributes are attributes that are specific to a particular instance of a class, while class attributes are attributes that are shared by all instances of a class. To check for an instance attribute, use the following syntax:
hasattr(object, "attribute_name")
To check for a class attribute, use the following syntax:
Read also:If I Have Ureaplasma Does My Partner Have It Too Signs Symptoms
hasattr(class, "attribute_name")
The `hasattr()` function is a useful tool for checking if an object has a specific attribute before trying to access it. This can help to avoid errors and make your code more robust.
Here are some examples of how to use the `hasattr()` function:
# Check if an object has a specific instance attributeif hasattr(object, "attribute_name"): # Do something# Check if an object has a specific class attributeif hasattr(class, "attribute_name"): # Do something
Python Check If Has Attribute
When working with objects in Python, it is often necessary to check if an object has a specific attribute. The `hasattr()` function can be used to perform this check.
- Object: The object to be checked.
- Attribute: The name of the attribute to be checked for.
- Return value: True if the object has the specified attribute, False otherwise.
Here are some examples of how the `hasattr()` function can be used:
- To check if an object has a specific instance attribute:
if hasattr(object, "attribute_name"): # Do somethingTo check if an object has a specific class attribute:
if hasattr(class, "attribute_name"): # Do something
The `hasattr()` function is a useful tool for checking if an object has a specific attribute before trying to access it. This can help to avoid errors and make your code more robust.
1. Object
When using the `hasattr()` function in Python, the first argument is the object to be checked. This can be any type of object, including instances of classes, modules, and built-in types.
Read also:Discover The Leading American Truck Manufacturers In The Industry
- Instances of classes: When checking if an instance of a class has a specific attribute, the object to be checked will be the instance itself.
- Modules: When checking if a module has a specific attribute, the object to be checked will be the module object.
- Built-in types: When checking if a built-in type has a specific attribute, the object to be checked will be the type object.
Here are some examples of how to use the `hasattr()` function to check if an object has a specific attribute:
- To check if an instance of a class has a specific attribute:
if hasattr(object, "attribute_name"): # Do somethingTo check if a module has a specific attribute:
if hasattr(module, "attribute_name"): # Do somethingTo check if a built-in type has a specific attribute:
if hasattr(type, "attribute_name"): # Do something
The `hasattr()` function is a useful tool for checking if an object has a specific attribute before trying to access it. This can help to avoid errors and make your code more robust.
2. Attribute
In Python, the `hasattr()` function is used to check if an object has a specific attribute. The second argument to the `hasattr()` function is the name of the attribute to be checked for.
- Attribute name: The attribute name can be any valid Python identifier. It can be a simple name, such as "name", or a dotted name, such as "module.class.attribute".
- Attribute type: The attribute can be any type of object, including instances of classes, modules, and built-in types.
- Attribute existence: The `hasattr()` function will return True if the object has the specified attribute, and False otherwise.
The `hasattr()` function is a useful tool for checking if an object has a specific attribute before trying to access it. This can help to avoid errors and make your code more robust.
3. Return value
The `hasattr()` function in Python checks if an object has a specific attribute and returns a boolean value accordingly. Understanding the return value is crucial in effectively utilizing the `hasattr()` function.
- Attribute Existence Check: The primary purpose of `hasattr()` is to determine whether an object possesses a particular attribute. If the object has the specified attribute, the function returns `True`, indicating its existence. Conversely, if the attribute is not found, it returns `False`. This boolean response allows developers to make informed decisions based on the attribute's presence or absence.
- Dynamic Attribute Inspection: `hasattr()` enables dynamic attribute inspection during runtime. It can be used to introspect an object's attributes without prior knowledge or hard-coding. This flexibility is particularly useful when working with dynamically generated objects or when dealing with complex object hierarchies.
- Error Prevention: By checking for attribute existence before accessing it, `hasattr()` helps prevent errors and exceptions. Attempting to access a non-existent attribute can lead to `AttributeError`, which can be avoided by using `hasattr()` to verify the attribute's presence beforehand.
- Code Robustness: Utilizing `hasattr()` enhances code robustness by handling situations where attributes may be missing or dynamically changing. It allows developers to write code that adapts to varying attribute availability, making it more resilient and less prone to errors.
In summary, the return value of `hasattr()` is central to its functionality. It provides a clear indication of whether an object has a specific attribute, enabling developers to make informed decisions, prevent errors, and write more robust and flexible code.
4. To check if an object has a specific instance attribute
When working with objects in Python, it is important to be able to check if an object has a specific instance attribute. This can be done using the `hasattr()` function. The `hasattr()` function takes two arguments: the object to be checked and the name of the attribute to be checked for. It returns `True` if the object has the specified attribute, and `False` otherwise.
- Facet 1: Checking for Instance Attributes
Instance attributes are attributes that are specific to a particular instance of a class. To check if an object has a specific instance attribute, you can use the following syntax:
if hasattr(object, "attribute_name"): # Do something
For example, the following code checks if the `person` object has an `age` attribute:
if hasattr(person, "age"): print(person.age)
- Facet 2: Avoiding Errors
Checking for instance attributes before accessing them can help to avoid errors. For example, the following code will raise an `AttributeError` if the `person` object does not have an `age` attribute:
print(person.age)
However, the following code will not raise an error, because it first checks if the `person` object has an `age` attribute before accessing it:
if hasattr(person, "age"): print(person.age)
- Facet 3: Dynamic Attribute Inspection
The `hasattr()` function can also be used to perform dynamic attribute inspection. This means that you can use the `hasattr()` function to check if an object has a specific attribute, even if you do not know the name of the attribute at compile time. For example, the following code checks if the `person` object has an attribute named `age` or `name`:
if hasattr(person, "age") or hasattr(person, "name"): # Do something
- Facet 4: Class Attributes vs. Instance Attributes
It is important to note that the `hasattr()` function checks for instance attributes, not class attributes. Class attributes are attributes that are shared by all instances of a class. To check if a class has a specific class attribute, you can use the following syntax:
if hasattr(class, "attribute_name"): # Do something
The `hasattr()` function is a versatile tool that can be used to check for both instance attributes and class attributes. It is a useful tool for avoiding errors and for performing dynamic attribute inspection.
5. To check if an object has a specific class attribute
The "To check if an object has a specific class attribute" aspect is tightly intertwined with "python check if has attribute" as it delves into the realm of examining attributes associated with classes rather than instances. This distinction is crucial for understanding the full capabilities of the `hasattr()` function and its versatility in working with Python objects.
- Facet 1: Class Attributes vs. Instance Attributes
Class attributes, unlike instance attributes, are shared among all instances of a class. They define common characteristics or properties that apply to every object created from that class. To check if an object possesses a specific class attribute, the syntax is as follows:
if hasattr(class, "attribute_name"): # Do something
For instance, consider a `Person` class with a class attribute `species` set to "Homo sapiens". Using `hasattr()`, we can verify this attribute's presence:
if hasattr(Person, "species"): print(Person.species) # Output: "Homo sapiens"
- Facet 2: Real-World Application
In real-world scenarios, checking for class attributes proves useful when dealing with shared properties across multiple instances. For example, in a database application, a `User` class may have a class attribute `default_privileges` that specifies the common permissions granted to all users. By leveraging `hasattr()`, we can dynamically inspect and modify these privileges as needed:
if hasattr(User, "default_privileges"): User.default_privileges.append("new_privilege")
- Facet 3: Error Handling
Similar to instance attributes, checking for class attributes before accessing them helps prevent errors. Attempting to access a non-existent class attribute directly can lead to `AttributeError`. Using `hasattr()` as a precautionary measure ensures code robustness and prevents unexpected program crashes:
if hasattr(User, "non_existent_attribute"): # Handle the absence of the attribute gracefully
- Facet 4: Dynamic Inspection and Reflection
Beyond error handling, `hasattr()` facilitates dynamic inspection and reflection of class attributes. It allows for runtime introspection of an object's attributes, enabling developers to adapt their code based on the availability of specific attributes. This flexibility is particularly valuable in scenarios where class attributes may vary or are generated dynamically.
In conclusion, understanding the distinction between class attributes and instance attributes is essential for effectively utilizing the `hasattr()` function. By embracing the facets discussed above, developers can harness the full potential of Python's attribute inspection capabilities, leading to more robust, adaptable, and error-resilient code.
Frequently Asked Questions about "python check if has attribute"
This section addresses common questions and misconceptions surrounding the usage of "python check if has attribute" to provide a comprehensive understanding of the topic.
Question 1: What is the primary purpose of the "python check if has attribute" functionality?The "python check if has attribute" functionality, implemented through the `hasattr()` function, serves the critical purpose of verifying the existence of a specific attribute associated with a given object. This attribute can be either an instance attribute, unique to a particular object instance, or a class attribute, shared among all instances of a class.
Question 2: How can I determine if an object possesses a specific attribute using Python?To ascertain whether an object has a particular attribute, Python offers the `hasattr()` function. This function takes two arguments: the object to be inspected and the name of the attribute in question. If the attribute exists, `hasattr()` returns `True`; otherwise, it returns `False`.
Question 3: What are the key benefits of employing the "python check if has attribute" approach?Utilizing the "python check if has attribute" approach offers several advantages. Firstly, it allows for graceful handling of scenarios where an attribute may be absent, preventing potential errors or exceptions. Secondly, it promotes code robustness by enabling dynamic attribute inspection, adapting to situations where attributes may be added or removed at runtime.
Question 4: How does "python check if has attribute" contribute to error prevention in Python programming?The "python check if has attribute" approach plays a crucial role in error prevention by allowing developers to verify the existence of an attribute before attempting to access it. This proactive measure helps avoid `AttributeError` exceptions, which can arise when accessing non-existent attributes, leading to more stable and reliable code.
Question 5: What are some real-world applications of the "python check if has attribute" functionality?The "python check if has attribute" functionality finds applications in various real-world scenarios. For instance, in object-oriented programming, it can be used to dynamically inspect class attributes, ensuring the availability of specific properties or methods before performing operations. Additionally, it proves useful in data validation, verifying the presence of required attributes within complex data structures.
Question 6: How does the "python check if has attribute" approach align with best practices in Python development?The "python check if has attribute" approach aligns closely with recommended best practices in Python development. By incorporating this practice, developers can write code that is more robust, adaptable, and less prone to errors. It promotes a proactive approach to attribute handling, emphasizing code quality and maintainability.
In summary, the "python check if has attribute" functionality is a versatile tool that empowers Python programmers to meticulously inspect objects and their attributes. By leveraging this approach, developers can enhance the robustness, reliability, and maintainability of their code.
Transition to the next article section...
Tips for Effectively Using "python check if has attribute"
The "python check if has attribute" approach, facilitated by the `hasattr()` function, provides a powerful mechanism for inspecting and verifying the presence of attributes associated with objects. To harness the full potential of this functionality, consider the following tips:
Tip 1: Embrace Proactive Attribute Verification
By actively checking for the existence of attributes before accessing them, you can prevent errors and exceptions, enhancing the robustness and stability of your code.
Tip 2: Leverage Dynamic Attribute Inspection
Utilize the `hasattr()` function to dynamically inspect objects and their attributes, adapting your code to handle scenarios where attributes may be added or removed at runtime.
Tip 3: Distinguish Between Instance and Class Attributes
Understand the distinction between instance attributes, unique to specific object instances, and class attributes, shared among all instances of a class. This knowledge ensures accurate attribute verification.
Tip 4: Enhance Code Readability and Maintainability
Incorporating the "python check if has attribute" approach into your code improves readability and maintainability by explicitly verifying the availability of attributes before use.
Tip 5: Foster Code Reusability and Flexibility
Employing the `hasattr()` function promotes code reusability and flexibility, allowing you to write code that can adapt to varying attribute availability or dynamically changing object structures.
Tip 6: Adhere to Pythonic Best Practices
Adopting the "python check if has attribute" approach aligns with Pythonic best practices, emphasizing code quality, reliability, and adherence to established coding conventions.
In summary, by incorporating these tips into your Python development practices, you can harness the full potential of the "python check if has attribute" functionality, leading to more robust, adaptable, and maintainable code.
Transition to the article's conclusion...
Conclusion
In conclusion, the "python check if has attribute" functionality, powered by the `hasattr()` function, provides a crucial mechanism for inspecting and verifying the presence of attributes associated with objects. By leveraging this approach, Python developers can enhance the robustness, reliability, and maintainability of their code.
The "python check if has attribute" approach empowers developers to proactively handle attribute availability, preventing errors and exceptions. It promotes dynamic attribute inspection, adapting to evolving object structures and attribute availability at runtime. Additionally, it fosters code reusability and flexibility, enabling the creation of code that can adapt to varying attribute availability and changing object structures.
Incorporating the "python check if has attribute" approach into Python development practices aligns with Pythonic best practices, emphasizing code quality, reliability, and adherence to established coding conventions. By embracing this approach, developers can write more robust, adaptable, and maintainable code, contributing to the overall health and longevity of their software applications.
Access Your MyHR Kohl's Anytime, Anywhere
Discover The Best Of Galena, IL At Best Western!
Discover The Secrets To Unlocking Dual Citizenship For Filipinos In Los Angeles
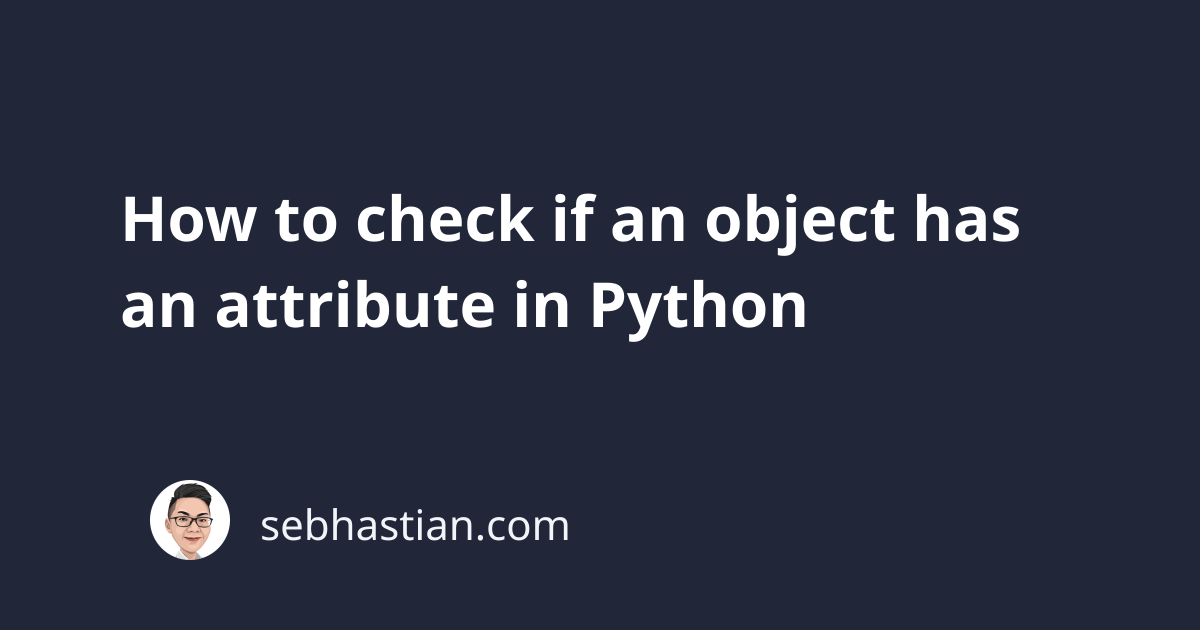
How to check if an object has an attribute in Python sebhastian

Python Check if Attribute Exists in Object with hasattr() Function

Check object has an attribute in Python Spark By {Examples}